Introduction to Python
Many Opportunities for python developer , lucrative salary and in demand skill.
Python is a high level programming level which is very usable for programming basic task with less effort, which makes it more compact and economical language to learn. Much Lower lines of code compared to Java,C or C++. Developed by Guido Van Rossum in 1991 , Open source community is still growing.
Why neccessary to Learn
- Simple to Learn
- Career Opportunity — Startup, large MNC all are using python for various uses like ML, Database , Analytics, Security, Mobile Dev, Web Scrapping etc
- Large Open Source Community — Latest updates and support
- Reliable and Efficient — Many Domains are using it , support for a large applications
- Extensive Library — Provide a Multitude of packages for various specific dev, Like matplotlib for visualization , pandas for data manipulation, pygame for game development , jango for web development etc
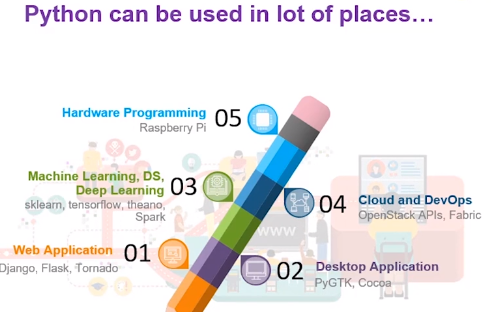
Installing of Python
- Download and Install Anaconda https://www.anaconda.com/distribution/ (avoid pip install for necessary DS packages ; and Installs Python too)
- Start using Jupyter notebook — basic but effective Notebook for python prg
Getting Started With Python
Python Variable
Unlike java, there is no need to specify the datatype of the variableWays of Assigning value to a variable
- Single value variable
a=10
name = 'Victor'
salary = 2000.23
print(a)
print(name)
print(salary)
-----------------------------------------------o/p:
10
Victor
2000.23
2. Multiple Assignment
#Assigning multiple value to a variable...
a=b=c=10
x,y,z=10,20,30
print(y)
print(a)
--------------------------------------------------op:
20
10
Python Token
It is the most basic unit of the source code

- Keyword — these are special reserved words that have specific meaning and function Ex: If, import, for …
- Identifier- it is the name that is used to identify function, variable or class . Rules are as follows 1. No special characters except ( _ ) 2. No keywords can be used. 3. Case sensitive. 4.can start with (_) ,or character but not digit
- Literals — Constants or data/value given to a variable

Literals Type
1.String Literal — Both single and double quotes are allowed
name1="John"
name2="James"
print(name1)
print(name2)
text1 ='hello\
world'
text1
multiline = '''str1
str2
str3'''o/p: John
James

2. Numeric Literal- In python , value of number is not restricted by the number of bits and can expand. Also no need for additional indication for long numbers
3. Boolean Literal — True and False
4. Special Literal — None (like null in java)
val1 = 10
val2 = None
val1,val2
print(val1,val2)
o/P:
10 None
IV. OPERATORS in Python

- Arithmetic Operator — takes two operands. Operations: +,-,*,/,%,**
- Assignment Operator =,+=, -=, *=
a=10
a*=10 #a=10*10
print(a)
o/p:
100
3. Comparison Operator <, >, <=, >=, !=
a = 10
b = 20
a > bo/P:
False
Logical Operator: and, or , not
a=10<10 and 2>-1
print(a)
o/P:
False
Bitwise Operator &,|,>>,<<,~
print(7 | 5)
print(7 & 5)
o/p:
7
5>>> 10>>2
2
>>> 10<<2
40
>>> 10<<4
80
Identity Operator is, is not
x = 10
x is 10
o/p: Truex = 10
x is not 10
o/P:
False
Membership Operator in, not in
pets=['dog','cat','wolf']
print('lion' in pets)
print('wolf' in pets)
'me' in 'appointmento/P : False
True
True
DataTypes in python

- Numbers
message = “Hello World”
num = 1234
pi = 13.6
print(type(message)) #return a string
print(type(num)) #return an integer
print(type(pi)) #return a floato/p:
<class 'str'>
<class 'int'>
<class 'float'>
2. Strings
var1 = 'Hello-World!'
var2 = 'PythonTutorial'print(var1[0]) # prints the first character in the string `H`
print(var2[1:5]) # prints the substring 'ytho' [inclusive:exclusive]
o/P:
H
ytho #find() – Returns the position of the string
str='Attachment'
str.find('Me')
o/p: 6#replace() – Replaces one character/string with other
str='Replacement'
str.replace('me','e')
o/p: Replaceent
str.replace('ent','')
o/p: Replacem#split() – Creates a split on the basis of a character
str='word1,word2,word3'
str.split(',')
o/p: [word1,word2,word3]#count() - count the number of occurance, case sensitive
str3 = "Intelligent"
str3.count("i")
o/p:1str4 = str3.upper()
str4.count("I")
o/p: 2
#max/min - returns max/min ascii value character from string
str5 = '!@#123AbcC'
max(str5) o/p: c
min(str5) o/p: !
3.tuples — values are inside (), since it is immutable data types values cannot be changed
#Concatenation - Adds two string/character
mytuple1 = ('a','b','c','d')
mytuple2 = ('e','f')
mytuple1+=mytuple2
print(mytuple1)
o/p:
('a','b','c','d','e','f')#Repetition - Duplicate string/character by given number of times
myGroup = ('a', 'b', 'c', 'd')
myGroup * 2
o/p: ('a',b','c','d','a','b','c','d')#Indexing - Shows the indexed character/string
myGroup[2]
o/p: 'c'myGroup[1:5]
o/p: 'a','b',c','d'#reversing shorthand
a=myGroup[::-1]
a
o/p: 'd','c','b','a'
4.List — group of mutable data types in [ ]
#Concatenation – Add elements to the list
myList = ['a', 1, 3.14, 'python']
myList+=['d',]
print(myList)o/p: ['a', 1, 3.14, 'python', 'd']#Remove
myList.remove('d')
myList
o/p: ['a', 1, 3.14, 'python']#append
myList.append(['a','b'])
myList.append('z')
myList
o/p: ['a', 1, 3.14, 'python', ['a','b'],'z']#extend
#myList.extend([1,2])
myList
o/p: ['a', 1, 3.14, 'python', ['a','b'],'z',1,2]#insert(index, value)
myList = ['a', 1, 3.14, 'python']
myList.insert(2, 'd') #insert after 2nd position
myList
o/p:['a', 1, 'd', 3.14, 'python']
myList.insert(2, ['a','b'])
myList
o/p: ['a', 1, ['a', 'b'], 'd', 3.14, 'python']#Repetition
myList = ['a', 1, 3.14, 'python']
myList*2
o/p: ['a', 1, 3.14, 'python', 'a', 1, 3.14, 'python']#Slicing
myList = ['a', 1, 3.14, 'python']
myList = myList[1:3] #(starting inclusive: ending exclusive)
myList
o/p: [1,3.14]
5. Dictionary — key, value pairs. Values in {} . we define an index for each variable
#empty dictionary
myDict = {}#dictionary with integer keys
myDict = {1: 'apple', 2: 'ball'}#dictionary with mixed keys
myDict = {'name': 'John', 1: [2, 4, 3]}#paring
#from sequence having each item as a pair
myDict = dict([(1,'apple'), (2,'ball')])
myDict
o/p: {1: 'apple', 2: 'ball'}#accessing dictionary
myDict = {1: 'word1', 2: 'word2'}
myDict[1]
o/p: 'word1'#len()
myDict = {1: 'word1', 2: 'word2'}
len(myDict)
o/p: 2#key()
myDict = {1: 'apple', 2: 'ball'}
myDict.keys()
o/p: dict_keys([1, 2])#values()
myDict = {1: 'apple', 2: 'ball'}
myDict.values()
o/p: ['apple', 'ball']#update
myDict = {1: 'apple', 2: 'ball'}
myDict.update({3: 'cat'})
print(myDict)myDict2 =({4:'dog'})
myDict.update(myDict2)
print(myDict)
o/p:
{1: 'apple', 2: 'ball', 3: 'cat'}
{1: 'apple', 2: 'ball', 3: 'cat', 4: 'dog'}
6. Sets — Inordered Collection of items in {}, doesnt have keys
#Creating set
mySet = {1, 2, 3, 3}
print (mySet)#Union
myS1 = {1, 2, 'c'}
myS2 = {1, 'b', 'c'}
myS1 | myS2
o/p: {1,2,'b','c'}#Intersection
>>>myS1 = {1, 2, 'c'}
>>>myS2 = {1, 'b', 'c'}
>>>myS1 & myS2
{1, 'c'}#Difference
>>>myS1 = {1, 2, 'c'}
>>>myS2 = {1, 'b', 'c'}
>>>myS1 - myS2
{2}
FlowControl
Indentation is very important
#If Statements can have and , or
a = 33
b = 200
if b > a:
print("b is greater than a")o/p: b is greater than a#elif and else
a = 36
b = 33
if b > a: #<-- false
print("b is greater than a")
elif a == b: #<-- false
print("a and b are equal")
else: #<-- else is executed when all if and elif condition fails
print("a is greater than b")o/p: a and b are equal#Nested if
x = 41
if x > 10:
print("Above ten,")
if x > 20:
print("and also above 20!")
else:
print("but not above 20.")
o.p: Above ten,
and also above 20!
While
while loop we can execute a set of statements as long as a condition is true.
a = 1
while a<4:
if(a%2==0):
print(a, 'is even')
else:
print(a, 'is odd')
a+=1
o/p: 1 is odd
2 is even
3 is odd# With the break statement we can stop the loop even if the while # condition is true:
i = 1
while i < 6:
print(i)
if i == 3:
break
i += 1
o/p: 1
2
3# With the continue statement we can stop the current iteration,
# and continue with the next:
i = 0
while i < 6:
i += 1
if i == 3:
continue
print(i)
o/p: 1
2
4
5
6
For — A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string)
#for example
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
o/p: apple
banana
cherry#looping through string
for x in "banana":
print(x)
o/p: b
a
n
a
n
a#break
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
if x == "banana":
break
o/p:
apple
banana#continue
fruits = ["apple", "banana", "cherry"]
for x in fruits:
if x == "banana":
continue
print(x)
o/p:
apple
cherryprint("case1")
for x in range(6):
print(x)print("case2")
for x in range(2, 6):
print(x)
print("case3")
for x in range(2, 30, 3):
print(x)
o/p:case1
0
1
2
3
4
5
case2
2
3
4
5
case3
2
5
8
11
14
17
20
23
26
29
Functions
A function is block of organized, reusable set of instructions that is used to perform some related action. we need them for reusability
Types of Functions
- User Defined function — functions defined by user
#Syntax
def func_name (arg1, arg2, arg3, ….):
statements…
return [expression]
def add (a,b):
sum = a + b
return sumadd(1,20)
o/p:21
2. Built in Function — Set of predefined functions built in python . Ex: abs(), all(), any(), ascii(),bin(),bool()
Calling Function
- pass by value — caller and callee have two independent variables
a=10
def ChangeIt(b):
print('value of b is', b)
b=100
print('New value of b is', b)ChangeIt(a)
print(a)
o/p:value of b is 10
New value of b is 100
10 #<--- value of parameter wasnt changeddef fun(a):
return a+10
b=fun(10)
print(b)
o/p: 20
2. pass by reference- caller and callee have same variable
c=[10,20,30]
def ChangeThem(d):
print('value of d is', d)
d[0]=99
d[1]=98
print('New value of d is', d)
ChangeThem(c)
print(c)
o/p:
value of d is [10, 20, 30]
New value of d is [99, 98, 30]
[99, 98, 30] #<-- list values were changed
Lambda Function- Anonymous function , can take any number of arguments but can have only one expression
lambda arguments : expression
x = lambda a : a + 10
print(x(5))
o/p:15r = lambda x,y:x*y
print(r(3,2))
o/p:6def myfunc(n):
return lambda a:a*n
d =myfunc(3)
print(d(2))
print(d(4))
d=myfunc(5)
print(d(4))
o/p:
6
12
20
Scope and nested Functions
Scope- part of the program where an object or name may be accessible
Types-
- global scope — defined in the main body of script, accessible generally everywhere in script
- local scope- defined inside the function,cannot be accessed outside the function
- Built in scope -names in the predefined built-in modules
Global vs local scope
#case 1 -Whenever we call variable in global scope it always #searches in global scope then builtin but never local scope#case 2- Whenever we call variable in local scope it always #searches in local scope then global then builtindef square(val):
#local scope
new_val = val**2
return new_val#global scope
new_val =10
print(square(new_val))new_val =10
print(square(new_val))# --> global variables are fetched at the #time of execution and not at the time of declarationo/p: 100
400
Global Keyword: If we want to create/change the value of a global variable
new_val =10
def square(val):
global new_val
new_val=new_val**2
return new_valprint(square(new_val))
print(new_val)o/p:
100
100
Scopes in Nested Functions
- Enclosing scope — in below example fn outer is enclosing function
- local scope — in below example inner is local scope, variable x would be first found in inner scope, if not found then enclosing scope,global scope and then builtin scope
- nonlocal keyword— in Nested Function to create/change for variable name in local scope
# Define echo_shout()
def echo_shout(word):
"""Change the value of a nonlocal variable"""
# Concatenate word with itself: echo_word
echo_word=word*2
# Print echo_word
print(echo_word)
# Define inner function shout()
def shout():
"""Alter a variable in the enclosing scope"""
# Use echo_word in nonlocal scope
nonlocal echo_word
# Change echo_word to echo_word concatenated with '!!!'
echo_word = echo_word+'!!!'
# Call function shout()
shout()
# Print echo_word
print(echo_word)# Call function echo_shout() with argument 'hello'
echo_shout('hello')o/p:
hellohello
hellohello!!!
Uses of Nested Functions
#1.scalability of functionality
def mod2plus6(a,b,c):
''' doc strings for documentation'''
def inner(x):
return x%2+6
return (inner(a),inner(b),inner(c)) #indentation is very impprint(mod2plus6(1,2,2))#2.Flexibility in Returning Functions - closure a bit difficult to read but code can be written as below
def raise_val(n):
def inner(x):
raised=x**n
return raised
return innerSquare=raise_val(2)
cube=raise_val(3)
print(Square(3),cube(3))o/p:
(7, 6, 6)
9 27
*args and **kwargs in Function
- *args allows you to do is take in zero or more arguments than the number of formal arguments
- ** kwargs as being a dictionary that maps each keyword to the value that we pass alongside it.
# case 1 -Function *args parameter
def myFun(arg1, *argv):
print ("First argument :", arg1)
for arg in argv:
print("Next argument through *argv :", arg)
myFun('Hello', 'Welcome', 'to', 'Python')o/p:
First argument : Hello
Next argument through *argv : Welcome
Next argument through *argv : to
Next argument through *argv : Python#case 2- Function *kwargs parameter
def myFun(arg1, **kwargs):
for key, value in kwargs.items():
print ("%s == %s" %(key, value))
myFun("Hi", first ='destiny', mid ='can be', last='changed')
o/P:
first == destiny
mid == can be
last == changed#case 3- sending variables as *args and **kwargs to function parameters
#def myFun(*argv): doesnt work in this case
def myFun(arg1,arg2,arg3):
print("arg1:", arg1)
print("arg2:", arg2)
print("arg3:", arg3)
# Now we can use *args or **kwargs to
# pass arguments to this function :
args = ("Karma", "is", "real")
myFun(*args)
kwargs = {"arg1" : "Karma", "arg2" : "is", "arg3" : "real"}
myFun(**kwargs)
o/P:
arg1: Karma
arg2: is
arg3: real
arg1: Karma
arg2: is
arg3: real
File Handling
- Open -
f = open (“path of the file”,mode)

#Example
myfile = open("C:/Users/mosa0816/Desktop/newTXT.txt","w")
#myfile.write("Ican write one more line")
myfile.write("Test............")
myfile1 = open("C:/Users/mosa0816/Desktop/newTXT.txt","r")
for x in myfile1:
print(x)
o/p: Test............
2. Read
#Example
f = open("C:/Users/mosa0816/Desktop/nexTXT1.txt", "r")
print(f.read())
o/p:
Hello World
This is a great day, Enjoy!#Reading parts of file
file="C:/Users/mosa0816/Desktop/nexTXT1.txt"
f = open(file, "r")
print(f.read(5)) #Return the 5 first characters of the file
o/p: Hello #Reading first line
f = open(file, "r")
print(f.readline()) #readline() is used to return one line
o/p: Hello World#Reading first line
f = open(file, "r")
print(f.readline())
print(f.readline()) # using readline() twice prints first two line
o/p: Hello World
This is a great day, Enjoy!
3. Write/Create
#Example: Append
f = open("demofile.txt", "a")
f.write("Now the file has one more line!")
o/p: 31 #<-- no of characters written#Example: Overwrite
f = open("demofile.txt", "w") #w - writes from start unlike a mode
f.write("Woops! I have deleted the content!")
o/p: 34
4. Delete
# Deleting the file
import os
os.remove("demofile.txt")